Your cart is currently empty!
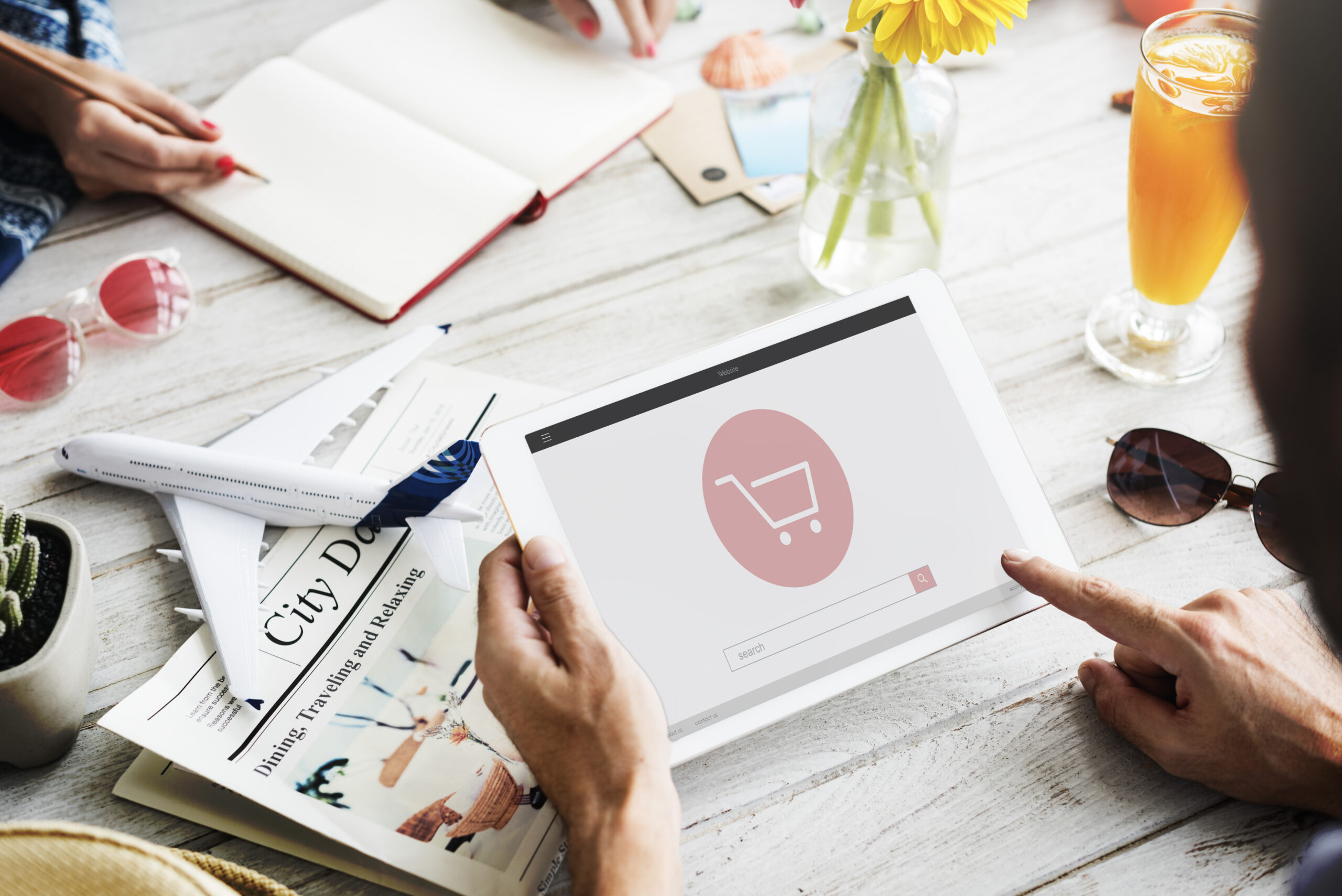
How to enable product downloads in WooCommerce for a custom order status
To enable product downloads in WooCommerce for a custom order status, you’ll need to use custom code.
By default, WooCommerce allows product downloads for orders in specific statuses like “Completed” or “Processing“. To extend this to include a custom status, you’ll modify the wc_customer_download_permissions filter to add your status.
Here’s how you can do it:
Steps to Enable Product Downloads in Custom Status
- Create Your Custom Order Status (if not already done): Use a snippet to add a custom order status in WooCommerce.
- Grant Download Permissions for the Custom Status: Add a filter to allow downloads for the custom status.
Code Snippet
// Add custom status to WooCommerce download permissions
add_filter('woocommerce_order_is_download_permitted', 'allow_download_for_custom_status', 10, 2);
function allow_download_for_custom_status($is_download_permitted, $order) {
// Replace 'your_custom_status' with your actual custom status slug
if ($order->get_status() === 'your_custom_status') {
$is_download_permitted = true;
}
return $is_download_permitted;
}
Steps to Use the Code:
- Add this code to your theme’s
functions.php
file or use a custom plugin. - Replace
your_custom_status
with the slug of your custom status (e.g.,pending-download
).
Verify the Custom Status Slug
To verify your custom status slug:
- Go to the WooCommerce > Orders section in your WordPress admin.
- Look for the order in your custom status and note its status slug from the URL or order details.
Testing
- Place a test order and set it to your custom status.
- Confirm that the downloadable products in that order are now accessible for the customer.
This customization ensures that downloads are enabled when an order reaches your custom-defined status.